Using Callback Functions to Respond to User Interface Events
- Updated2023-02-21
- 4 minute(s) read
Using Callback Functions to Respond to User Interface Events
Callback functions respond to all events generated by the User Interface Library. The C prototypes for the callback functions are in userint.h. You can assign callback functions to panels, menu bars, controls, or the main callback. When the user generates an event on a particular user interface object, the appropriate callback function executes. Idle events and end-task events are passed to the main callback function only. InstallMainCallback installs this main callback function.
LabWindows/CVI passes event information from the GUI to the callback functions. For example, callback functions receive the type of user interface event that occurred, such as EVENT_LEFT_CLICK, and some additional information concerning that event, such as the x- and y-coordinates of the mouse cursor when the click occurred. You are free to design your functions to use this information when responding to events. LabWindows/CVI also passes callback data to the callback function that you define.
If you want to swallow an event, make sure that the callback function returns 1. Otherwise, callback functions must return 0.
A top-level panel callback receives the EVENT_CLOSE message callback when the user selects System»Close or clicks the Close button in the upper right corner of the panel title bar.
A panel callback receives the EVENT_PANEL_SIZE message when a user or the program changes the size of a panel.
![]() |
Note If ATTR_DISABLE_PROG_PANEL_SIZE_EVENTS is set to true, you cannot programmatically change the size of a panel. |
A panel callback receives the EVENT_PANEL_MOVE message when a user moves a panel.
The main callback receives the EVENT_END_TASK message when the user tries to shut down Windows or when the user tries to terminate the application.
The tutorial in Getting Started with LabWindows/CVI and many of the sample programs that are packaged with LabWindows/CVI demonstrate how to use callback functions. The following diagram and pseudocode also illustrate this concept.
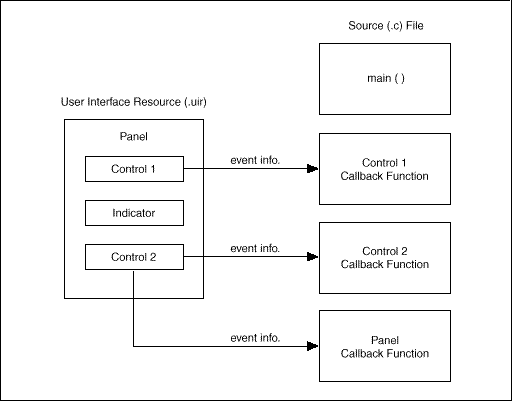
Callback Function Concept
panel_handle = LoadPanel(...);
DisplayPanel(panel_handle);
menu_handle = LoadMenuBar(...);
RunUserInterface();
int CVICALLBACK PanelResponse (int handle, int event, void *callbackdata, int eventdata1, int eventdata2)
{
switch (event) {
case EVENT_PANEL_SIZE :
. /* Code that responds to the panel */
. /* being resized */
break;
case EVENT_PANEL_MOVE :
. /* Code that responds to the panel */
. /* being moved */
break;
case EVENT_KEYPRESS :
. /* Code that responds to a keypress */
. /* eventdata1 & eventdata2 contain */
/* keycode information */
break;
}
return(0);
}
int CVICALLBACK ControlResponse (int handle, int control, int event, void *callbackdata, int eventdata1, int eventdata2)
{
if (control == PANEL_CONTROL1) {
switch (event) {
case EVENT_RIGHT_CLICK :
. /* Code that responds to a right */
. /* click on CONTROL1 */
break;
case EVENT_VAL_CHANGED :
. /* Code that responds to a value */
. /* change on CONTROL1 */
break;
case EVENT_COMMIT :
. /* Code that responds to a commit */
. /* event on CONTROL1 */
break;
}
}
if (control == PANEL_CONTROL2) {
switch (event) {
case EVENT_RIGHT_CLICK :
. /* Code that responds to a right */
. /* click on CONTROL2 */
break;
case EVENT_COMMIT :
. /* Code that responds to a commit */
. /* event on CONTROL2 */
break;
}
}
return(0);
}
int CVICALLBACK MenuBarResponse (int menubar, int menuitem, void *callbackdata, int panel)
{
switch (menuitem) {
case MENUBAR_MENU1_ITEM1:
. /* Code that responds to ITEM1 in */
. /* MENU1 of the menu bar. */
break;
case MENUBAR_MENU1_ITEM2:
. /* Code that responds to ITEM2 in */
. /* MENU1 of the menu bar. */
break;
}
return(0);
}
![]() |
Note If you assign callback functions to your GUI objects using the User Interface Editor, LoadPanel and LoadMenuBar automatically install these functions. Otherwise, you must install them programmatically through the callback installation functions (InstallPanelCallback, InstallCtrlCallback, InstallMenuCallback, and others). |
![]() |
Note Do not call longjmp from within a callback function. |
The CVICALLBACK macro should precede the function name in the declarations and function headers for all user interface callbacks. This ensures that the functions are treated by the compiler as cdecl, even when the default calling convention is stdcall. CVICALLBACK is defined in cvidef.h, which is included by userint.h. The CVICALLBACK macro is included where necessary in the header files generated by the User Interface Editor and in source code generated by CodeBuilder.