Tutorial: Implementing Hello World in OpenMP
- Updated2023-02-21
- 2 minute(s) read
Tutorial: Implementing Hello World in OpenMP
OpenMP allows you to easily create applications that execute on multiple threads with minimal re-writing of existing code. This tutorial walks you through parallelizing the standard Hello World application using OpenMP. The following code is typical code for a Hello World application.
#include <ansi_c.h>
#include <stdio.h>
int main (int argc, char *argv[])
{
printf("Hello World\n");
printf("Press the Enter key to exit.");
getchar ();
}
Complete the following steps to parallelize the Hello World application using OpenMP.
- After creating a project and opening a source file, enable OpenMP support for your project by selecting Enable OpenMP support in the Build Options dialog box.
- Copy the Open World code above into the source file.
- Include the OpenMP library for compilation:
#include <omp.h>
#include <ansi_c.h>
#include <stdio.h>
- Add code that allows OpenMP applications to compile in older versions of LabWindows/CVI that do not support OpenMP:
#ifdef _OPENMP
#include <omp.h>
#endif
#include <ansi_c.h>
#include <stdio.h>
- Create a region of code designated to execute, in parallel, on multiple threads:
int main (int argc, char *argv[])
{
#pragma omp parallel
{
printf("Hello World\n");
}
printf("Press the Enter key to exit.");
getchar ();
}
- Update the code to display which threads execute the parallel region:
int main (int argc, char *argv[])
{
#pragma omp parallel
{
printf("Hello World from thread = %d\n", omp_get_thread_num());
}
printf("Press the Enter key to exit.");
getchar ();
}
- Add code to specify only the master thread displays how many threads are available in the team:
int main (int argc, char *argv[])
{
#pragma omp parallel
{
printf("Hello World from thread = %d\n", omp_get_thread_num());
#pragma omp master
printf("Number of threads in the team = %d\n", omp_get_num_threads());
}
printf("Press the Enter key to exit.");
getchar ();
}
In the previous exercise, you used used OpenMP to create a parallel region using the parallel directive. Some applications you want to update using OpenMP will be more complex and you might want to share data and communicate between threads in a team or need to use synchronization constructs to avoid data conflicts and race conditions. This help file provides basic conceptual information related to these topics; however, consider exploring comprehensive OpenMP resources for more in-depth information.
The following image represents the complete, commented source code for the Hello World application updated with the OpenMP structure.
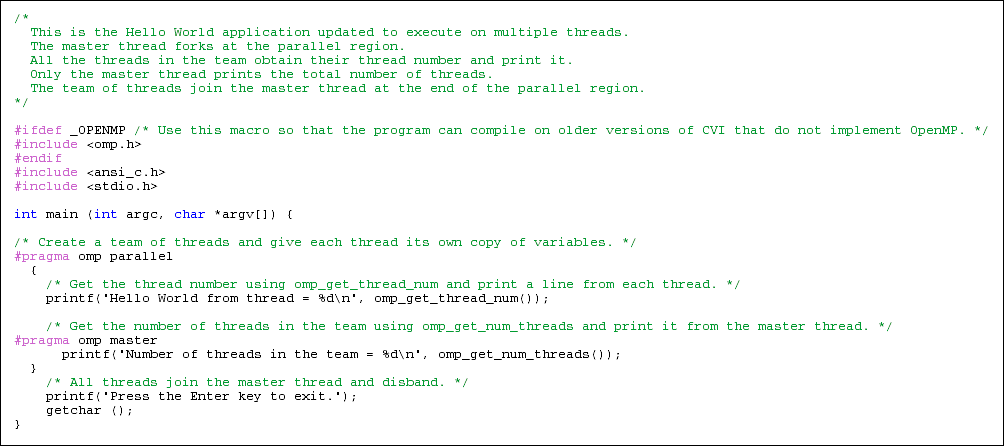
Related Topics
OpenMP Specification Compliance
OpenMP Compiler Directives and Clauses
Communicating between Threads and Sharing Data